Situation
I have this custom layout for a tableView cell that has two UITextView
inside of the cell. If you just start using voice over it will select one of the two UITextView
and then read each individual line within as shown below.
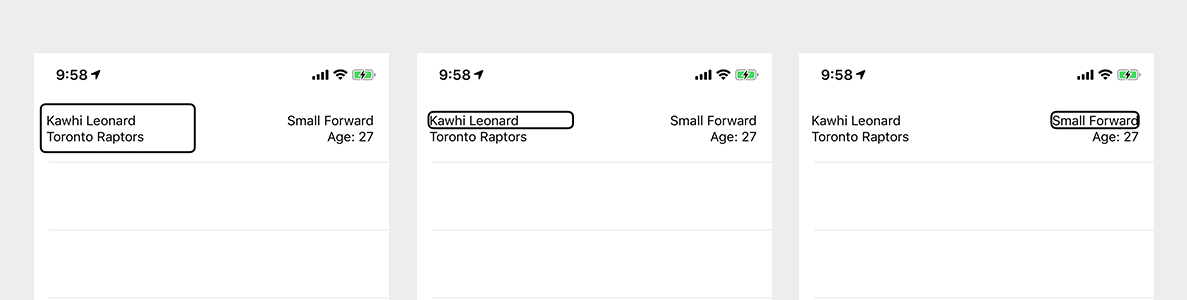
When you start interacting with your interface with voice over turned on you realize that it is not ideal for this type of navigation.
Ideal Situation
Instead what I would like is to ignore the 2 textViews within the cell and have the entire tableView cell selectable. And then read it out in a full sentence.
I have omitted a lot of the boiler plate code for setting up tableViews. The real work happens inside of HomeTableViewCell
.
class HomeTableViewController: UITableViewController {
var model = [
Player(name: "Kawhi Leonard", team: "Toronto Raptors", position: "Small Forward", age: "27")
]
...
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath) as! HomeTableViewCell
let player = model[indexPath.row]
// Configure the cell
cell.configure(with: player)
return cell
}
}
Voice Over for TableView Cell
Within my configure()
method I ignored the 2 UITextView
within the cell. Made the tableView cell itself be selectable. And then have it read it out in a full sentence by concating some of those variables. See code below.
class HomeTableViewCell: UITableViewCell {
@IBOutlet var leftTextView: UITextView!
@IBOutlet var rightTextView: UITextView!
func configure(with player: Player) {
// This is what appears in the tableView cell
self.leftTextView.text = "\(player.name)\n\(player.team)"
self.rightTextView.text = "\(player.position)\n Age: \(player.age)"
// 1. Make Voice over ignore the UITextView
self.leftTextView.isAccessibilityElement = false
self.rightTextView.isAccessibilityElement = false
// 2. Make Voice Over highlight this UITableViewCell as an element
isAccessibilityElement = true
// 3. Improve the read back by customizing the label. This should sound much nicer.
accessibilityLabel = "\(player.name) is a player for \(player.team). Plays the position of \(player.position). \(player.age) years old"
accessibilityHint = "Double tap to get more details"
}
}
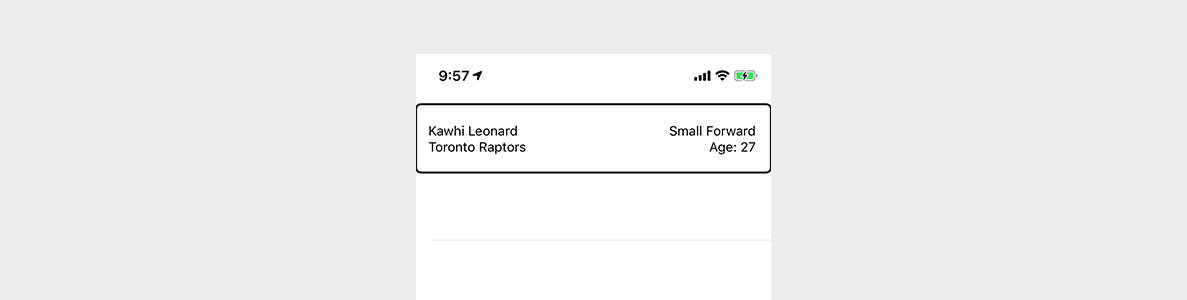
Now when I test my app, the entire row is selectable. It reads the row as a full sentence.
"Kawahi Leonard is a player for Toronto Raptors. Plays the position of small forward. 27 years old"
(PAUSES)
"Double tap to get more details"
Conclusion
Always be testing your interface within Voice Over. You don’t know if your interface is good enough until you do.
The next design exercise would be changes to the tableViewCell based on the users preferred content size. Apple has some api’s that can make it easy to change out the tableView cell from two column layout to a single column layout.
I will post an other article on how to adapt to that.